14.8.2. steps.API_1.geom
Namespace for geometry objects.
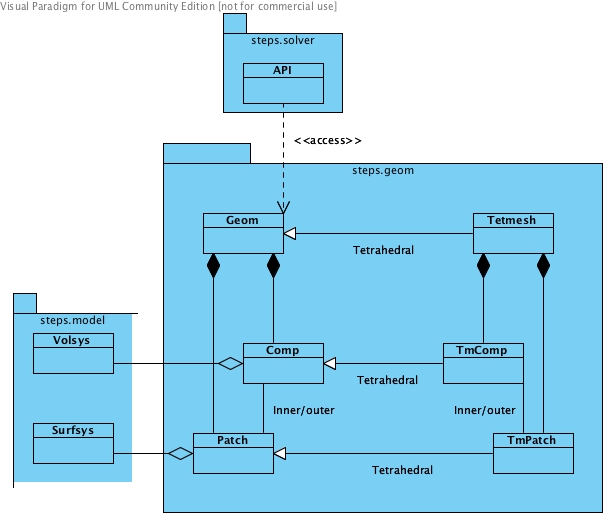
Class diagram for steps.API_1.geom namespace.
- class Geom[source]
Top-level geometry container to which a number of compartment objects and patches objects may be grouped.
- A steps.geom.Geom object is parent to the following objects:
steps.geom.Comp
steps.geom.Patch
Construction:
g = steps.geom.Geom()
Create a geometry container object.
Arguments: None
- delComp(id)
Removes the steps.geom.Comp object with identifier string comp_id (if defined) from the geometry container.
Syntax:
delComp(comp_id)
Arguments: string comp_id
Return: None
- delPatch(id)
Removes the steps.geom.Patch object with identifier string patch_id (if defined) from the geometry container.
Syntax:
delPatch(patch_id)
Arguments: string patch_id
Return: None
- getAllComps()
Returns a list of references to all steps.geom.Comp compartment objects in the geometry container.
Syntax:
getAllComps()
Arguments: None
Return: list<steps.geom.Comp>
- getAllPatches()
Returns a list of references to all steps.geom.Patch patch objects in the geometry container.
Syntax:
getAllPatches()
Arguments: None
Return: list<steps.geom.Patch>
- getComp(id)
Returns a reference to the steps.model.Comp compartment object with identifier string comp_id (if defined).
Syntax:
getComp(comp_id)
Arguments: string comp_id
Return: steps.model.Comp
- getPatch(id)
Removes the steps.geom.Patch object with identifier string patch_id (if defined) from the geometry container.
Syntax:
getPatch(patch_id)
Arguments: string patch_id
Return: steps.geom.Patch
- this
An identification of the underlying cpp object
- class Comp(id, container, vol=0)[source]
Base class for compartment objects. It provides basic functionality and data that is shared by the derived class steps.geom.TmComp (that is used to describe a compartment in a tetrahedral mesh):
Getting and setting a valid compartment identifier string, and handling the interaction with the container object.
Getting (and at least in this base class also setting) the total volume of the compartment.
Adding volume systems to the compartment.
References to steps.geom.Patch objects to which the compartment is adjoined.
This base class can be used directly with well-mixed solvers.
Relationship between Compartments and Patches
It is necessary to explain the inner/outer relationship between compartments and patches. When a patch object is created (a surface of a compartment, which may be shared with another compartment). it is necessary to arbitrarily label the compartment(s) “inner” and “outer” (if a patch is connected to only one compartment then the compartment must be labelled “inner” by convention). This is necessary in order to fully describe the surface reaction rules. Accordingly, compartments also store a list of connections, “inner” patches and “outer” patches. So if a patch1 is created with comp1 as it’s “inner” compartment, comp1 knows patch 1 as an “outer” patch. The labelling is purely defined when creating the Patch objects, bearing in mind the stoichiometry defined in the surface reaction objects. This may seem a little confusing at first, but will become clearer when experience is gained with these objects.
See also
Surface-Volume Reactions (Example: IP3 Model)
Construction:
comp = steps.geom.Comp(id, container, vol = 0.0)
Construct a compartment object with identifier string id and assign container as the parent geometry container. Optionally set volume to vol (in m^3).
Arguments: string id steps.geom.Geom container float vol (default = 0.0)
- addVolsys(id)
Add volume system identifier string volsys_id to the compartment object.
Syntax:
addVolsys(volsys_id)
Arguments: string volsys_id
Return: None
- property container
Reference to parent steps.geom.Geom container.
- delVolsys(id)
Removes volume system identifier string volsys_id from this compartment.
Syntax:
delVolsys(volsys_id)
Arguments: string volsys_id
Return: None
- getAllDiffs(model)
Given a steps.model.Model, return all diffusions in the compartment.
Syntax:
getAllDiffs(model)
Arguments: steps.model.Model model
Return: list<steps.model.Diff>
- getAllReacs(model)
Given a steps.model.Model, return all reactions in the compartment.
Syntax:
getAllReacs(model)
Arguments: steps.model.Model model
Return: list<steps.model.Reac>
- getAllSpecs(model)
Given a steps.model.Model, return all species in the compartment. That is, all species that appear in the compartment due to volume system reaction and diffusion rules, plus surface system surface reactions, voltage-dependent surface reactions etc where reactants or products reference this compartment as ‘inner’ or ‘outer’ compartment.
Syntax:
getAllSpecs(model)
Arguments: steps.model.Model model
Return: list<steps.model.Spec>
- getContainer()
Returns a reference to the parent steps.geom.Geom container object.
Syntax:
getContainer()
Arguments: None
Return: steps.geom.Geom
- getID()
Get the identifier string of the compartment.
Syntax:
getID()
Arguments: None
Return: string
- getIPatches()
Returns a list of references to steps.geom.Patch patch objects: the ‘inner’ patches.
Syntax:
getIPatches()
Arguments: None
Return: list<steps.geom.Patch>
- getOPatches()
Returns a list of references to steps.geom.Patch patch objects: the ‘outer’ patches.
Syntax:
getOPatches()
Arguments: None
Return: list<steps.geom.Patch>
- getVol()
Get the volume of the compartment (in m^3).
Syntax:
getVol()
Arguments: None
Return: float
- getVolsys()
Returns a list of the volume system identifier strings which have been added to the compartment.
Syntax:
getVolsys()
Arguments: None
Return: list<string>
- property id
Identifier string of the compartment.
- property ipatches
List of reference to inner patches.
- property opatches
List of reference to outer patches.
- setID(id)
Set the identifier string of the compartment.
Syntax:
setID(name)
Arguments: string name
Return: None
- setVol(vol)
Set the volume of the compartment (in m^3).
Syntax:
setVol(vol)
Arguments: float vol
Return: None
- this
An identification of the underlying cpp object
- property vol
Volume of the compartment.
- property volsys
Reference to assocated volume system.
- class Patch(id, container, icomp, area=0)[source]
Base class for patch objects. A patch is a piece of 2D surface surrounding (part of) a 3D compartment, which may be connected to another compartment. It provides basic functionality and descriptive data that is shared by the derived class steps.geom.TmPatch (that is used to describe a surface comprised of triangles in a tetrahedral mesh):
Getting and setting a valid patch identifier string, and handling the interaction with the container object.
Getting (and at least in this base class also setting) the total area of the patch.
Adding surface systems to the patch.
References to inside/outside compartments to which the patch is adjoined.
This base class can be used directly with well-mixed solvers.
Construction:
patch = steps.geom.Patch(id, container, icomp, ocomp = None, area = 0.0)
Construct a patch object with identifier string id, assign container as the parent geometry container and assign icomp as the “inner” compartment. Assign also ocomp as the “outer” compartment (if required) and optionally set the area to area (in m^2).
Arguments: string id steps.geom.Geom container steps.geom.Comp icomp steps.geom.Comp ocomp (default = None) float area (default = 0.0)
Note
“Inner” compartment and “outer” compartment are purely defined by their order to the class constructor.
- addSurfsys(id)
Add surface system identifier string surfsys_id to the patch object.
Syntax:
addSurfsys(surfsys_id)
Arguments: string surfsys_id
Return: None
- property area
Area of the patch.
- property container
Reference to parent steps.geom.Geom container.
- delSurfsys(id)
Removes surface system identifier string surfsys_id from this patch.
Syntax:
delSurfsys(surfsys_id)
Arguments: string surfsys_id
Return: None
- getAllSReacs(model)
Given a steps.model.Model, return all surface reactions in the compartment.
Syntax:
getAllSReacs(model)
Arguments: steps.model.Model model
Return: list<steps.model.SReac>
- getAllSpecs(model)
Given a steps.model.Model, return all species in the patch. That is, all ‘surface’ species referenced in surface system surface reactions, voltage-dependent surface reactions and surface diffusion. This also includes ohmic current and ghk current ‘channel states’.
Syntax:
getAllSpecs(model)
Arguments: steps.model.Model model
Return: list<steps.model.Spec>
- getArea()
Get the area of the patch (in m^2).
Syntax:
getArea()
Arguments: None
Return: float
- getContainer()
Returns a reference to the parent steps.geom.Geom container object.
Syntax:
getContainer()
Arguments: None
Return: steps.geom.Geom
- getIComp()
Returns a reference to the steps.geom.Comp compartment object representing the inner compartment.
Syntax:
getIComp()
Arguments: None
Return: steps.geom.Comp
- getID()
Get the identifier string of the patch.
Syntax:
getID()
Arguments: None
Return: string
- getOComp()
Returns a reference to the steps.geom.Comp compartment object representing the outer compartment.
Syntax:
getOComp()
Arguments: None
Return: steps.geom.Comp
- getSurfsys()
Returns a list of the surface system identifier strings which have been added to the patch.
Syntax:
getSurfsys()
Arguments: None
Return: list<string>
- property icomp
Reference to the inner compartment.
- property id
Identifier string of the patch.
- property ocomp
Reference to the outer compartment.
- setArea(vol)
Set the area of the patch (in m^2).
Syntax:
setArea(area)
Arguments: float area
Return: None
- setID(id)
Set the identifier string of the patch.
Syntax:
setID(name)
Arguments: string name
Return: None
- property surfsys
Reference to assocated surface system.
- this
An identification of the underlying cpp object
- class Tetmesh(verts, tets, tris=[])[source]
Main container class for static tetrahedral meshes. This class stores the vertices points, 3D tetrahedral and 2D triangular elements that comprise the mesh. The indices of the elements will be stored as unsigned integers (a positive integer or zero) beginning at zero and incremented by 1. For example, if there are ntets number of tetrahedrons in the mesh, the indices of the tetrahedrons will be [0,1,2,…, (ntets-1)]. In addition, this class computes and contains some auxiliary data from the mesh:
Rectangular, axis-aligned bounding box.
Overall volume.
The total number of tetrahedrons in the mesh.
The total number of triangles in the mesh.
The total number of vertices in the mesh.
Auxiliary data is also stored for each tetrahedron:
Volume of the tetrahedron.
Indices of the 4 neighbouring tetrahedrons. If there is no neighbour (i.e. if the tetrahedron lies on the border), this index will be UNKNOWN_TET. The sequence of neighbours is determined by the following common boundary triangles: (0,1,2), (0,1,3), (0,2,3), (1,2,3).
Indices of the 4 neighbouring boundary triangles. The sequence of neighbours is also determined by (0,1,2), (0,1,3), (0,2,3), (1,2,3).
Compartment (steps.geom.TmComp object) that the tetrahedron belongs to. Stores zero pointer if the tetrahedron has not been assigned to a compartment.
And for each triangle:
Area of the triangle.
Normal vector of the triangle, normalized to length 1.0.
Indices of the 2 neighbouring tetrahedrons. If one tetrahedron does not exist (because the triangle lies on the outer boundary), this index will be UNKNOWN_TET.
Patch (steps.geom.TmPatch object) that a triangle belongs to. Stores zero pointer if triangle has not been assigned to a patch.
Syntax:
mesh = steps.geom.Tetmesh(verts, tets, tris)
Construct a Tetmesh container: Supply a list of all vertices verts (by Cartesian coordinates), supply a list of all tetrahedrons tets (by indices of the 4 vertices) and supply a full or partial list of triangles tris (by indices of the 3 vertices). Indexing in STEPS begins at zero, so the first 3 coordinates in verts will describe the zeroth vertex, t he next 3 coordinates will describe the 1st vertex and so on. Labelling of the vertices in tets and tris should follow this indexing. Lists must be one-dimensional. Length of verts = nverts*3 where nverts is the total number of vertices; length of tets = ntets*4 where ntets is the total number of tetrahedrons; maximum length of tris ntris*3 where ntris is the total number of triangles. For example, if we have just three tetrahedrons; tet0=[0,1,2,3], tet1=[0,1,3,4] and tet2=[1,3,4,5] then the required one-dimensional list tets=[0,1,2,3,0,1,3,4,1,3,4,5].
Arguments: list<double> verts list<index_t> tets list<index_t> tris
- addROI(id, type, indices)
Add a Region of Interest data record with name id to the ROI dataset. The type of elements stored in the ROI data can be one of the follows: steps.geom.ELEM_VERTEX, steps.geom.ELEM_TET, steps.geom.ELEM_TRI, steps.geom.ELEM_UNDEFINED.
Syntax:
addROI(id, type, indices)
Arguments: string id ElementType type set<index_t> indices
Return: None
- checkROI(id, type, count=0, warning=True)
Check if an ROI enquire is valid.
Syntax:
checkROI(id, type, count=0, warning=true)
Arguments: string id ElementType type int count bool warning
Return: bool
- countBars()
Returns the total number of bars in the mesh.
Syntax:
countBars()
Arguments: None
Return: int
- countTets()
Returns the total number of tetrahedrons in the mesh.
Syntax:
countTets()
Arguments: None
Return: int
- countTris()
Returns the total number of triangles in the mesh.
Syntax:
countTris()
Arguments: None
Return: int
- countVertices()
Returns the total number of vertices in the mesh.
Syntax:
countVertices()
Arguments: None
Return: int
- delComp(id)
Removes the steps.geom.Comp object with identifier string comp_id (if defined) from the geometry container.
Syntax:
delComp(comp_id)
Arguments: string comp_id
Return: None
- delPatch(id)
Removes the steps.geom.Patch object with identifier string patch_id (if defined) from the geometry container.
Syntax:
delPatch(patch_id)
Arguments: string patch_id
Return: None
- findTetByPoint(p)
Returns the index of the tetrahedron which encompasses a given point p (given in Cartesian coordinates x,y,z). Returns -1 if p is a position outside the mesh. It uses either findTetByPointLinear or findTetByPointWalk depending on the mesh size.
Syntax:
findTetByPoint(p)
Arguments: list<float, length = 3> p
Return: index_t
- findTetByPointLinear(p)
Returns the index of the tetrahedron which encompasses a given point p (given in Cartesian coordinates x,y,z). Returns -1 if p is a position outside the mesh. Linear search. Only suitable for small meshes.
Syntax:
findTetByPointLinear(p)
Arguments: list<double, length = 3> p
Return: index_t
- findTetByPointWalk(p)
Returns the index of the tetrahedron which encompasses a given point p (given in Cartesian coordinates x,y,z). Returns -1 if p is a position outside the mesh. A* search. After a seeding round of random points the algorithm walks towards the closest tetrahedron. It works for disconnected meshes too. Usually faster than a linear search for normal-sized meshes.
Syntax:
findTetByPointWalk(p)
Arguments: list<double, length = 3> p
Return: index_t
- genPointsInTet(tidx, npnts, coords)
Generate npnts random point coordinates x,y,z within a tetraedron with index tidx, export it to NumPy array cords
Syntax:
genPointsInTet(t_idx, npnts, coords)
Arguments: index_t tidx int npnts numpy.array<float, length = npnts * 3> coords
Return: None
- genPointsInTri(tidx, npnts, coords)
Generate npnts random point coordinates x,y,z within a triangle with index tidx, export it to NumPy array cords
Syntax:
genPointsInTri(t_idx, npnts, coords)
Arguments: index_t tidx int npnts numpy.array<float, length = npnts * 3> coords
Return: None
- genROITetVisualPointsNP(ROI_id, point_counts, coords)
For each tetrahedron index in a ROI, randomly generate a set of point coordinates x,y,z within the tetrahedron, where n is stored in point_counts. The number of points required to be generated for tetrahedron i in the ROI is point_counts[i]. All generated points are stored in cords.
Syntax:
genTetVisualPointsNP(ROI_id, point_counts, coords)
Arguments: string ROI_id numpy.array<uint> point_counts numpy.array<double> coords
Return: None
- genROITriVisualPointsNP(ROI_id, point_counts, coords)
For each triangle index in a ROI, randomly generate a set of point coordinates x,y,z within the triangle, where n is stored in point_counts. The number of points required to be generated for triangle i in the ROI is point_counts[i]. All generated points are stored in cords.
Syntax:
genROITriVisualPointsNP(ROI_id, point_counts, coords)
Arguments: string ROI_id numpy.array<uint> point_counts numpy.array<double> coords
Return: None
- genTetVisualPointsNP(indices, point_counts, coords)
For each tetrahedron index in indices, randomly generate a set of point coordinates x,y,z within the tetrahedron, where n is stored in point_counts. The number of points required to be generated for tetrahedron indices[i] is point_counts[i]. All generated points are stored in cords.
Syntax:
genTetVisualPointsNP(indices, point_counts, coords)
Arguments: numpy.array<index_t> indices numpy.array<uint, length = length(indices)> point_counts numpy.array<double, length = sum(point_counts) * 3> coords
Return: None
- genTriVisualPointsNP(indices, point_counts, coords)
For each triangle index in indices, randomly generate a set of point coordinates x,y,z within the triangle, where n is stored in point_counts. The number of points required to be generated for triangle indices[i] is point_counts[i]. All generated points are stored in cords.
Syntax:
genTriVisualPointsNP(indices, point_counts, coords)
Arguments: numpy.array<index_t> indices numpy.array<uint, length = length(indices)> point_counts numpy.array<double, length = sum(point_counts) * 3> coords
Return: None
- getAllComps()
Returns a list of references to all steps.geom.TmComp compartment objects in the tetmesh container.
Syntax:
getAllComps()
Arguments: None
Return: list<steps.geom.TmComp>
- getAllPatches()
Returns a list of references to all steps.geom.TmPatch patch objects in the tetmesh container.
Syntax:
getAllPatches()
Arguments: None
Return: list<steps.geom.TmPatch>
- getAllROINames()
Get a list of the names of all Region of Interest data stored in ROI dataset.
Syntax:
getAllROINames()
Arguments: None
Return: list<string>
- getBar(bidx)
Returns the vertices of bar with index bidx in the container.
Syntax:
getBar(bidx)
Arguments: index_t bidx
Return: list<index_t, length = 2>
- getBatchTetVolsNP(indices, volumes)
Get the volumes of a list of tetrahedrons in indices and stored in volumes.
Syntax:
getBatchTetVolsNP(indices, volumes)
Arguments: numpy.array<index_t> indices numpy.array<double, length = length(indices)> volumes
Return: None
- getBatchTets(tets)
Get vertex indices of a list of tetrahedrons.
Syntax:
getBatchTets(tets)
Arguments: list<index_t> tets
Return: list<index_t, length = len(tets) * 4>
- getBatchTetsNP(t_indices, v_indices)
Get vertex indices of a list of triangles.
Syntax:
getBatchTetsNP(t_indices, v_indices)
Arguments: numpy.array<index_t> t_indices numpy.array<index_t, length = len(t_indices) * 4> v_indices
Return: None
- getBatchTriAreasNP(indices, areas)
Get the areas of a list of triangles in indices and stored in areas.
Syntax:
getBatchTriAreasNP(indices, areas)
Arguments: numpy.array<index_t> indices numpy.array<float, length = length(indices)> areas
Return: None
- getBatchTris(tris)
Get vertex indices of a list of triangles.
Syntax:
getBatchTris(tris)
Arguments: list<index_t> tris
Return: list<index_t, length = len(tris) * 3>
- getBatchTrisNP(t_indices, v_indices)
Get vertex indices of a list of triangles.
Syntax:
getBatchTrisNP(t_indices, v_indices)
Arguments: numpy.array<index_t> t_indices numpy.array<index_t, length = len(t_indices) * 3> v_indices
Return: None
- getBatchVertices(verts)
Get coordinates of a list of vertices.
Syntax:
getBatchVertices(verts)
Arguments: list<index_t> verts
Return: list<float, length = len(verts) * 3>
- getBatchVerticesNP(indices, coordinates)
Get coordinates of a list of vertices.
Syntax:
import numpy as np indices = np.array([0, 1, 2], dtype= np.uint64) coordinates = np.zeros(len(indices) * 3) getBatchVertices(indices, coordinates)
Arguments: numpy.array<index_t> indices numpy.array<float, length = len(indices) * 3> coordinates
Return: None
- getBoundMax()
Returns the maximal Cartesian coordinate of the rectangular bounding box of the mesh.
Syntax:
getBoundMax()
Arguments: None
Return: list<float, length = 3>
- getBoundMin()
Returns the minimal Cartesian coordinate of the rectangular bounding box of the mesh.
Syntax:
getBoundMin()
Arguments: None
Return: list<float, length = 3>
- getComp(id)
Returns a reference to the steps.model.Comp compartment object with identifier string comp_id (if defined).
Syntax:
getComp(comp_id)
Arguments: string comp_id
Return: steps.model.Comp
- getMeshVolume()
Returns the total volume of the mesh.
Syntax:
getMeshVolume()
Arguments: None
Return: float
- getNROIs()
Get the number of Region of Interest data stored in the ROI dataset.
Syntax:
getNROIs()
Arguments: None
Return: int
- getPatch(id)
Removes the steps.geom.Patch object with identifier string patch_id (if defined) from the geometry container.
Syntax:
getPatch(patch_id)
Arguments: string patch_id
Return: steps.geom.Patch
- getROI(id)
Get a Region of Interest data record with name id.
Syntax:
getROI(id)
Arguments: string id
Return: ROISet
- getROIArea(ROI_id)
Get summed area of all triangles stored in a triangular ROI.
Syntax:
getROIArea(id)
Arguments: string id
Return: float
- getROIData(id)
Get the stored data of a Region of Interest data record with name id.
Syntax:
getROIData(id)
Arguments: string id
Return: list<index_t>
- getROIDataSize(id)
Get the number of elements stored in a Region of Interest data record with name id.
Syntax:
getROIDataSize(id)
Arguments: string id
Return: int
- getROITetBarycenters(ROI_id)
Get barycenters of elements stored in a tetrahedral ROI.
Syntax:
getROITetBarycenters(ROI_id)
Arguments: string ROI_id
Return: list<float>
- getROITetBarycentersNP(ROI_id, centers)
Get barycenters of elements stored in a tetrahedral ROI and write to a NumPy array centers. The size of centers should be the same as the number of elements stored in the ROI.
Syntax:
getROITetBarycentersNP(ROI_id, centers)
Arguments: string ROI_id numpy.array<float> centers
Return: None
- getROITetBarycentres(ROI_id)
Get barycenters of elements stored in a tetrahedral ROI.
This function has been renamed by replacing “centre” with “center”. It will be deprecated in the next release.
Syntax:
getROITetBarycenters(ROI_id)
Arguments: string ROI_id
Return: list<float>
- getROITetBarycentresNP(ROI_id, centers)
Get barycenters of elements stored in a tetrahedral ROI and write to a NumPy array centers. The size of centers should be the same as the number of elements stored in the ROI.
This function has been renamed by replacing “centre” with “center”. It will be deprecated in the next release.
Syntax:
getROITetBarycentersNP(ROI_id, centers)
Arguments: string ROI_id numpy.array<float> centers
Return: None
- getROITetVerticesMappingSetNP(ROI_id, t_vertices, v_set)
Add all vertex indices of a list of tetrahedrons in a ROI to a set and write it to a NumPy array v_set. For each of the tetrahedron, t_vertices records the positions of its vertices in v_set. i.e. For the i tetrahedron in the ROI, the STEPS indices of its vertices are v_set[t_vertices[4*i]], v_set[t_vertices[4*i + 1]], v_set[t_vertices[4*i + 2]], v_set[t_vertices[4*i + 3]]
Syntax:
getROITetVerticesMappingSetNP(ROI_id, t_vertices, v_set)
Arguments: string ROI_id numpy.array<index_t> v_indices numpy.array<index_t> v_set
Return: None
- getROITetVerticesSetSizeNP(ROI_id)
Add all vertex indices of a list of tetrahedrons in a ROI to a set and return its size.
Syntax:
getROITetVerticesSetSizeNP(ROI_id)
Arguments: string ROI_id
Return: int
- getROITetVolsNP(ROI_id, volumes)
Get the volumes of a list of tetrahedrons in a ROI and stored in volumes.
Syntax:
getROITetVolsNP(indices, volumes)
Arguments: string ROI_id numpy.array<float> volumes
Return: None
- getROITets(ROI_id)
Get vertices of elements stored in a tetrahedral ROI.
Syntax:
getROITets(ROI_id)
Arguments: string ROI_id
Return: list<index_t>
- getROITetsNP(ROI_id, v_indices)
Get vertices of elements stored in a tetrahedral ROI and write to a NumPy array v_indices. The size of v_indices should be 3 * the number of elements stored in the ROI.
Syntax:
getROITetsNP(ROI_id, v_indices)
Arguments: string ROI_id numpy.array<index_t> v_indices
Return: None
- getROITriAreasNP(ROI_id, areas)
Get the areas of a list of triangles in a ROI and stored in areas.
Syntax:
getROITriAreasNP(indices, areas)
Arguments: string ROI_id numpy.array<float> areas
Return: None
- getROITriBarycenters(ROI_id)
Get barycenters of elements stored in a triangular ROI.
Syntax:
getROITriBarycenters(ROI_id)
Arguments: string ROI_id
Return: list<float>
- getROITriBarycentersNP(ROI_id, centers)
Get barycenters of elements stored in a triangular ROI and write to a NumPy array centers. The size of centers should be the same as the number of elements stored in the ROI.
Syntax:
getROITriBarycenters(ROI_id)
Arguments: string ROI_id
Return: list<float>
- getROITriBarycentres(ROI_id)
Get barycenters of elements stored in a triangular ROI.
This function has been renamed by replacing “centre” with “center”. It will be deprecated in the next release.
Syntax:
getROITriBarycenters(ROI_id)
Arguments: string ROI_id
Return: list<float>
- getROITriBarycentresNP(ROI_id, centers)
Get barycenters of elements stored in a triangular ROI and write to a NumPy array centers. The size of centers should be the same as the number of elements stored in the ROI.
This function has been renamed by replacing “centre” with “center”. It will be deprecated in the next release.
Syntax:
getROITriBarycentersNP(ROI_id, centers)
Arguments: string ROI_id numpy.array<float> centers
Return: None
- getROITriVerticesMappingSetNP(ROI_id, t_vertices, v_set)
Add all vertex indices of a list of triangles in a ROI to a set and write it to a NumPy array v_set. For each of the triangle, t_vertices records the positions of its vertices in v_set. i.e. For the i triangle in the ROI, the STEPS indices of its vertices are v_set[t_vertices[3*i]], v_set[t_vertices[3*i + 1]], v_set[t_vertices[3*i + 2]]
Syntax:
getROITriVerticesMappingSetNP(ROI_id, t_vertices, v_set)
Arguments: string ROI_id numpy.array<index_t> v_indices numpy.array<index_t> v_set
Return: None
- getROITriVerticesSetSizeNP(ROI_id)
Add all vertex indices of a list of triangles in a ROI to a set and return its size.
Syntax:
getROITriVerticesSetSizeNP(ROI_id)
Arguments: string ROI_id
Return: int
- getROITris(ROI_id)
Get vertices of elements stored in a triangular ROI.
Syntax:
getROITris(ROI_id)
Arguments: string ROI_id
Return: list<index_t>
- getROITrisNP(ROI_id, v_indices)
Get vertices of elements stored in a triangular ROI and write to a NumPy array v_indices. The size of v_indices should be 3 * the number of elements stored in the ROI.
Syntax:
getROITrisNP(ROI_id, v_indices)
Arguments: string ROI_id numpy.array<index_t> v_indices
Return: None
- getROIType(id)
Get the element type of a Region of Interest data record with name id.
Syntax:
getROIType(id)
Arguments: string id
Return: ElementType
- getROIVertices(ROI_id)
Get coordinates of elements stored in a vertices ROI.
Syntax:
getROIVertices(ROI_id)
Arguments: string ROI_id
Return: list<float>
- getROIVerticesNP(ROI_id, coordinates)
Get coordinates of elements stored in a vertices ROI and write to a NumPy array coordinates. The size of coordinates should be the same as the number of elements stored in the ROI.
Syntax:
getROIVerticesNP(ROI_id, coordinates)
Arguments: string ROI_id numpy.array<float> coordinates
Return: None
- getROIVol(ROI_id)
Get summed area of all tetrahedrons stored in a tetrahedral ROI.
Syntax:
getROIVol(id)
Arguments: string id
Return: float
- getSurfTris()
Returns a list of triangles that form the mesh boundary. Support function for steps.utilities.visual.
Syntax:
getTriBoundary()
Arguments: None
Return: list<index_t>
- getTet(tidx)
Returns the tetrahedron with index tidx in the container by its four vertex indices.
- Syntax::
getTet(tidx)
Arguments: index_t tidx
Return: list<index_t, length = 4>
- getTetBarycenter(tidx)
Returns the barycenter of the tetrahedron with index tidx.
Syntax:
getTetBarycenter(tidx)
Arguments: index_t tidx
Return: list<float, length = 3>
- getTetComp(tidx)
Returns a reference to a steps.geom.Comp object: the compartment which tetrahedron with index tidx belongs to. Returns None if tetrahedron not assigned to a compartment.
Syntax:
getTetComp(tidx)
Arguments: index_t tidx
Return: steps.geom.TmComp
- getTetQualityRER(tidx)
Returns the radius-edge-ratio (a quality measurement) of tetrahedron with index tidx.
Syntax:
getTetQualityRER(tidx)
Arguments: index_t tidx
Return: float
- getTetTetNeighb(tidx)
Returns the indices of the four neighbouring tetrahedrons of tetrahedron with index tidx. An index of -1 indicates no neighbour (tetrahedron is on the mesh border).
Syntax:
getTetTetNeighb(tidx)
Arguments: index_t tidx
Return: list<index_t, length = 4>
- getTetTriNeighb(tidx)
Returns the indices of the four neighbouring triangles of tetrahedron with index tidx.
Syntax:
getTetTriNeighb(tidx)
Arguments: index_t tidx
Return: list<index_t, length = 4>
- getTetVerticesMappingSetNP(t_indices, t_vertices, v_set)
Get the vertex indices of a list of tetrahedrons. The vertex indices are reindexed, with their oringinal STEPS indices stored in a given array, whose size is provided by getTriVerticesSetSizeNP().
Syntax:
getTetVerticesMappingSetNP(t_indices, t_vertices, v_set)
Arguments: numpy.array<index_t> t_indices numpy.array<index_t, length = length(t_indices) * 4> t_vertices numpy.array<index_t, length = getTriVerticesSetSizeNP(t_indices)> v_set
Return: None
- getTetVerticesSetSizeNP(t_indices)
Return the size of a set with unique vertex indices of a list of tetrahedrons, preparation function for furture numpy data access.
Syntax:
getTetVerticesSetSizeNP(t_indices)
Arguments: numpy.array<index_t> t_indices
Return: index_t
- getTetVol(tidx)
Returns the volume of the tetrahedron with index tidx.
Syntax:
getTetVol(tidx)
Arguments: index_t tidx
Return: float
- getTri(tidx)
Returns the triangle with index tidx in the container by its three vertex indices.
Syntax:
getTri(tidx)
Arguments: index_t tidx
Return: list<index_t, length = 3>
- getTriArea(tidx)
Returns the area of the triangle with index tidx.
Syntax:
getTriArea(tidx)
Arguments: index_t tidx
Return: float
- getTriBars(tidx)
Returns the index of the bars that comprise the triangle.
Syntax:
getTriBars(tidx)
Arguments: index_t tidx
Return: list<int, length = 3>
- getTriBarycenter(tidx)
Returns the Cartesian coordinates of the barycenter of triangle with index tidx.
Syntax:
getTriBarycenter(tidx)
Arguments: index_t tidx
Return: list<float, length = 3>
- getTriDiffBoundary(tidx)
Returns a reference to a step.geom.Diffboundary object: the diffusion boundary triangle with index tidx belongs to. Returns None if triangle not assigned to a diffusion boundary.
Syntax:
getTriDiffBoundary(tidx)
Arguments: index_t tidx
Return: steps.geom.DiffBoundary
- getTriNorm(tidx)
Returns the normal vector of the triangle with index tidx.
Syntax:
getTriNorm(tidx)
Arguments: index_t tidx
Return: list<float, length = 3>
- getTriPatch(tidx)
Returns a reference to a step.geom.TmPatch object: the patch which triangle with index tidx belongs to. Returns None if triangle not assigned to a patch.
Syntax:
getTriPatch(tidx)
Arguments: index_t tidx
Return: steps.geom.TmPatch
- getTriTetNeighb(tidx)
Returns the indices of the two neighbouring tetrahedrons of triangle with index tidx. An index of UNKNOWN_TET indicates no neighbour (triangle is on the mesh border).
Syntax:
getTriTetNeighb(tidx)
Arguments: index_t tidx
Return: list<index_t, length = 2>
- getTriTriNeighb(tidx, tmpatch)
Returns the indices of the neighbouring triangles (that is all triangles that share a ‘bar’) of triangle with index tidx within the same patch.
Syntax:
getTriTriNeighb(int)
Arguments: index_t tidx
Returns: list<index_t>
- getTriTriNeighbs(tidx)
Returns the indices of the neighbouring triangles (that is all triangles that share a ‘bar’) of triangle with index tidx.
Syntax:
getTriTriNeighbs(int)
Arguments: index_t tidx
Returns: list<index_t>
- getTriVerticesMappingSetNP(t_indices, t_vertices, v_set)
Get the vertex indices of a list of triangles. The vertex indices are reindexed, with their oringinal STEPS indices stored in a given array, whose size is provided by getTriVerticesSetSizeNP().
Syntax:
getTriVerticesMappingSetNP(t_indices, t_vertices, v_set)
Arguments: numpy.array<index_t> t_indices numpy.array<index_t, length = length(t_indices) * 3> t_vertices numpy.array<index_t, length = getTriVerticesSetSizeNP(t_indices)> v_set
Return: None
- getTriVerticesSetSizeNP(t_indices)
Return the size of a set with unique vertex indices of a list of triangles, preparation function for furture numpy data access.
Syntax:
getTriVerticesSetSizeNP(t_indices)
Arguments: numpy.array<index_t> t_indices
Return: int
- getVertex(vidx)
Returns the coordinates of vertex with index vidx in the container.
Syntax:
getVertex(vidx)
Arguments: index_t vidx
Return: list<float, length = 3>
- intersect(double[:, point_coords, sampling=-1)
Computes the intersection of line segment(s) given the vertices with the current mesh
- Args:
- points: A 2-D numpy array (/memview), where each position contains the 3 point
coordinates
- int sampling: not specified or sampling < 1 –> use deterministic method
sampling > 0 –> use montecarlo method with sampling points
- Returns:
A list where each position contains the list of intersected tets (and respective intersection ratio) of each line segment.
- intersectIndependentSegments(double[:, point_coords, sampling=-1)
Similar to the intersect method but here we deal with independent segments, i.e. every two points we have a segment not related to previous or following ones. E.g. seg0 = (points[0], points[1]), seg1 = (points[2], points[3]), etc.
- Args:
- points: A 2-D numpy array (/memview), where each position contains the 3 point
coordinates
- int sampling: not specified or sampling < 1 –> use deterministic method
sampling > 0 –> use montecarlo method with sampling points
- Returns:
A list where each position contains the list of intersected tets (and respective intersection ratio) of each line segment.
- isPointInTet(p, tidx)
Check if point belongs to the tetrahedron or not
Syntax:
isPointInTet(p, tidx)
Arguments: list<float, length = 3> p int tetrahedron tidx
Return: bool
- property ntets
Number of tetrahedrons in the mesh.
- property ntris
Number of triangles in the mesh.
- property nverts
Number of vertices in the mesh.
- reduceBatchTetPointCountsNP(indices, point_counts, max_density)
Reduce the number of random point coordinates generated for each tetrahedron in indices so that the point density of the tetrahedron is below max_density. If the density is already below max_density for that tetrahedron, the count stored in point_counts is intacted.
Syntax:
reduceBatchTetPointCountsNP(indices, point_counts, max_density)
Arguments: numpy.array<index_t> indices numpy.array<uint, length = length(indices)> point_counts float max_density
Return: None
- reduceBatchTriPointCountsNP(indices, point_counts, max_density)
Reduce the number of random point coordinates generated for each triangle in indices so that the point density of the triangle is below max_density. If the density is already below max_density for that triangle, the count stored in point_counts is intacted.
Syntax:
reduceBatchTriPointCountsNP(indices, point_counts, max_density)
Arguments: numpy.array<index_t> indices numpy.array<uint, length = length(indices)> point_counts double max_density
Return: None
- reduceROITetPointCountsNP(ROI_id, point_counts, max_density)
Reduce the number of random point coordinates generated for each tetrahedron in a ROI so that the point density of the tetrahedron is below max_density. If the density is already below max_density for that tetrahedron, the count stored in point_counts is intacted.
Syntax:
reduceROITetPointCountsNP(indices, point_counts, max_density)
Arguments: string ROI_id numpy.array<uint> point_counts double max_density
Return: None
- reduceROITriPointCountsNP(ROI_id, point_counts, max_density)
Reduce the number of random point coordinates generated for each triangle in a ROI so that the point density of the triangle is below max_density. If the density is already below max_density for that triangle, the count stored in point_counts is intacted.
Syntax:
reduceROITriPointCountsNP(ROI_id, point_counts, max_density)
Arguments: string ROI_id numpy.array<uint, length = length(indices)> point_counts double max_density
Return: None
- removeROI(id)
Remove a Region of Interest data record with name id.
Syntax:
removeROI(id)
Arguments: string id
Return: None
- replaceROI(id, type, indices)
Replace a Region of Interest data record with name id with new data.
Syntax:
replaceROI(id, type, indices)
Arguments: string id ElementType type set<index_t> indices
Return: None
- setTetComp(tidx, comp)
Set the compartment which tetrahedron with index tidx belongs to..
Syntax:
setTetComp(tidx, comp)
Arguments: index_t tidx steps.geom.TmComp comp
Return: None
- setTriDiffBoundary(tidx, diffb)
Set the diffusion boundary which triangle with index tidx belongs to..
Syntax:
setTriDiffBoundary(triangle_global_id(tidx), diffb)
Arguments: index_t tidx steps.geom.DiffBoundary diffb
Return: None
- setTriPatch(tidx, patch)
Set the patch which triangle with index tidx belongs to..
Syntax:
setTriPatch(tidx, patch)
Arguments: index_t tidx steps.geom.TmPatch patch
Return: None
- this
An identification of the underlying cpp object
- class TmComp(id, container, tets)[source]
Derived class from base steps.geom.Comp class. It provides the same functionality as the steps.geom.Comp class extended for annotation of a group of tetrahedrons in a Tetmesh. The volume is the total volume of the encapsulated tetrahedrons.
Construction:
tmcomp = steps.geom.Comp(id, container, tets)
Construct a TmComp object with identifier string id and assign container as the parent Tetmesh container. Set the group of tetrahedrons that describe this compartment with tets.
Arguments: string id steps.geom.Tetmesh container list<index_t> tets
- addVolsys(id)
Add volume system identifier string volsys_id to the compartment object.
Syntax:
addVolsys(volsys_id)
Arguments: string volsys_id
Return: None
- property container
Reference to parent steps.geom.Geom container.
- countTets()
Returns the number of tetrahedrons assigned to the compartment.
Syntax:
countTets()
Arguments: None
Return: int
- delVolsys(id)
Removes volume system identifier string volsys_id from this compartment.
Syntax:
delVolsys(volsys_id)
Arguments: string volsys_id
Return: None
- getAllDiffs(model)
Given a steps.model.Model, return all diffusions in the compartment.
Syntax:
getAllDiffs(model)
Arguments: steps.model.Model model
Return: list<steps.model.Diff>
- getAllReacs(model)
Given a steps.model.Model, return all reactions in the compartment.
Syntax:
getAllReacs(model)
Arguments: steps.model.Model model
Return: list<steps.model.Reac>
- getAllSpecs(model)
Given a steps.model.Model, return all species in the compartment. That is, all species that appear in the compartment due to volume system reaction and diffusion rules, plus surface system surface reactions, voltage-dependent surface reactions etc where reactants or products reference this compartment as ‘inner’ or ‘outer’ compartment.
Syntax:
getAllSpecs(model)
Arguments: steps.model.Model model
Return: list<steps.model.Spec>
- getAllTetIndices()
Returns a list of all tetrahedrons assigned to the compartment.
Syntax:
getAllTetIndices()
Arguments: None
Return: list<int>
- getBoundMax()
Returns the maximal Cartesian coordinate of the rectangular bounding box of the compartment.
Syntax:
getBoundMax()
Arguments: None
Return: list<float, length = 3>
- getBoundMin()
Returns the minimal Cartesian coordinate of the rectangular bounding box of the compartment.
Syntax:
getBoundMin()
Arguments: None
Return: list<float, length = 3>
- getContainer()
Returns a reference to the parent steps.geom.Geom container object.
Syntax:
getContainer()
Arguments: None
Return: steps.geom.Geom
- getID()
Get the identifier string of the compartment.
Syntax:
getID()
Arguments: None
Return: string
- getIPatches()
Returns a list of references to steps.geom.Patch patch objects: the ‘inner’ patches.
Syntax:
getIPatches()
Arguments: None
Return: list<steps.geom.Patch>
- getOPatches()
Returns a list of references to steps.geom.Patch patch objects: the ‘outer’ patches.
Syntax:
getOPatches()
Arguments: None
Return: list<steps.geom.Patch>
- getVol()
Get the volume of the compartment (in m^3).
Syntax:
getVol()
Arguments: None
Return: float
- getVolsys()
Returns a list of the volume system identifier strings which have been added to the compartment.
Syntax:
getVolsys()
Arguments: None
Return: list<string>
- property id
Identifier string of the compartment.
- property ipatches
List of reference to inner patches.
- isTetInside(tets)
Returns a list of Booleans describing if tetrahedrons tets are assigned to the compartment.
Syntax:
isTetInside(tets)
Arguments: list<index_t> tets
Return: list<bool, length = length(tets)>
- property opatches
List of reference to outer patches.
- setID(id)
Set the identifier string of the compartment.
Syntax:
setID(name)
Arguments: string name
Return: None
- setVol(vol)
Obsolete
- property tets
List of indices of tetrahedrons associated to the compartment.
- this
An identification of the underlying cpp object
- property vol
Volume of the compartment.
- property volsys
Reference to assocated volume system.
- class TmPatch(id, container, tris, icomp)[source]
Derived class from base steps.geom.Patch class. It provides the same functionality as the steps.geom.Patch class extended for annotation of a group of triangles in a Tetmesh. The area is the total area of the encapsulated triangles.
Construction:
tmpatch = steps.geom.Comp(id, container, tris, icomp, ocomp = None)
Construct a TmPatch object with identifier string id and assign container as the parent geometry container. Set the collection of triangles in the patch to tris and assign icomp as the “inner” compartment and assign also ocomp as the “outer” compartment (if required).
Arguments: string id steps.geom.Tetmesh container list<index_t> tris steps.geom.TmComp icomp steps.geom.TmComp ocomp (default = None)
- addSurfsys(id)
Add surface system identifier string surfsys_id to the patch object.
Syntax:
addSurfsys(surfsys_id)
Arguments: string surfsys_id
Return: None
- property area
Area of the patch.
- property container
Reference to parent steps.geom.Geom container.
- delSurfsys(id)
Removes surface system identifier string surfsys_id from this patch.
Syntax:
delSurfsys(surfsys_id)
Arguments: string surfsys_id
Return: None
- getAllEndocyticZones()
Get all endocytic zones declared in the patch
- Return type
List[EndocyticZone]
- getAllSReacs(model)
Given a steps.model.Model, return all surface reactions in the compartment.
Syntax:
getAllSReacs(model)
Arguments: steps.model.Model model
Return: list<steps.model.SReac>
- getAllSpecs(model)
Given a steps.model.Model, return all species in the patch. That is, all ‘surface’ species referenced in surface system surface reactions, voltage-dependent surface reactions and surface diffusion. This also includes ohmic current and ghk current ‘channel states’.
Syntax:
getAllSpecs(model)
Arguments: steps.model.Model model
Return: list<steps.model.Spec>
- getAllTriIndices()
Returns a list of indices of all triangles assigned to the patch.
Syntax:
getAllTriIndices()
Arguments: None
Return: list<index_t>
- getArea()
Get the area of the patch (in m^2).
Syntax:
getArea()
Arguments: None
Return: float
- getBoundMax()
Returns the maximal Cartesian coordinate of the rectangular bounding box of the compartment.
Syntax:
getBoundMax()
Arguments: None
Return: list<float, length = 3>
- getBoundMin()
Returns the minimal Cartesian coordinate of the rectangular bounding box of the compartment.
Syntax:
getBoundMin()
Arguments: None
Return: list<float, length = 3>
- getContainer()
Returns a reference to the parent steps.geom.Geom container object.
Syntax:
getContainer()
Arguments: None
Return: steps.geom.Geom
- getIComp()
Returns a reference to the steps.geom.Comp compartment object representing the inner compartment.
Syntax:
getIComp()
Arguments: None
Return: steps.geom.Comp
- getID()
Get the identifier string of the patch.
Syntax:
getID()
Arguments: None
Return: string
- getOComp()
Returns a reference to the steps.geom.Comp compartment object representing the outer compartment.
Syntax:
getOComp()
Arguments: None
Return: steps.geom.Comp
- getSurfsys()
Returns a list of the surface system identifier strings which have been added to the patch.
Syntax:
getSurfsys()
Arguments: None
Return: list<string>
- property icomp
Reference to the inner compartment.
- property id
Identifier string of the patch.
- isTriInside(tris)
Returns a list of Booleans describing if triangles tris are assigned to the patch.
Syntax:
isTriInside(tris)
Arguments: list<index_t> tris
Return: list<bool, length = length(tris)>
- property ocomp
Reference to the outer compartment.
- setArea(vol)
Set the area of the patch (in m^2).
Syntax:
setArea(area)
Arguments: float area
Return: None
- setID(id)
Set the identifier string of the patch.
Syntax:
setID(name)
Arguments: string name
Return: None
- property surfsys
Reference to assocated surface system.
- this
An identification of the underlying cpp object
- property tris
List of indices of triangles associated to the patch.
- class DiffBoundary(id, container, tris)[source]
Annotation of a group of triangles in a Tetmesh. The triangles form a boundary between two compartments, that may allow diffusion of some specified species.
Construction:
diffb = steps.geom.DiffBoundary(id, container, tris)
Construct a DiffBoundary object with identifier string id and assign container as the parent geometry container, described by group of triangles tris.
Arguments: string id steps.geom.Tetmesh container list<index_t> tris
- property comps
Reference to two steps.tetmesh.Comp compartments connected by this diffusion boundary.
- property container
Reference to parent steps.tetmesh.Tetmesh container.
- getAllTriIndices()
Returns a list of indices of all triangles assigned to the diffusion boundary.
Syntax:
getAllTriIndices()
Arguments: None
Return: list<index_t>
- getComps()
Returns a list of the two compartments this diffusion boundary connects.
Syntax:
getComps()
Arguments: None
Return: list<steps::wm::Comp, length = 2>
- getContainer()
Returns a reference to the parent steps.tetmesh.Tetmesh container object.
Syntax:
getContainer()
Arguments: None
Return: steps.tetmesh.Tetmesh
- getID()
Get the identifier string of the diffusion boundary.
Syntax:
getID()
Arguments: None
Return: string
- property id
Identifier string of the diffusion boundary.
- isTriInside(tri)
Returns a list of Booleans describing if triangles tris are assigned to the diffusion boundary.
Syntax:
isTriInside(tris)
Arguments: list<index_t> tris
Return: list<bool, length = length(tris)>
- setID(id)
Set the identifier string of the diffusion boundary.
Syntax:
setID(name)
Arguments: string name
Return: None
- this
An identification of the underlying cpp object
- property tris
List of indices of triangles associated to the diffusion boundary.
- class SDiffBoundary(id, container, bars, patches)[source]
Annotation of a group of bars in a Tetmesh. The bars form a boundary between two patches, that may allow diffusion of some specified species.
Construction:
sdiffb = steps.geom.SDiffBoundary(id, container, bars, patches)
Construct a SDiffBoundary object with identifier string id and assign container as the parent geometry container, described by group of bars. Specify the patches to be connected by this surface diffusion boundary to avoid potential ambiguity.
Arguments: string id steps.geom.Tetmesh container list<index_t> bars list<steps.geom.TmPatch> (length 2) patches
- property bars
List of indices of bars associated to the surface diffusion boundary.
- property container
Reference to parent steps.tetmesh.Tetmesh container.
- getAllBarIndices()
Returns a list of indices of all bars assigned to the surface diffusion boundary.
Syntax:
getAllBarIndices()
Arguments: None
Return: list<int>
- getContainer()
Get a reference to the parent steps.tetmesh.Tetmesh container object.
Syntax:
getContainer()
Arguments: None
Return: steps.tetmesh.Tetmesh
- getID()
Get the identifier string of the surface diffusion boundary.
Syntax:
getID()
Arguments: None
Return: string
- getPatches()
Returns a list of the two patches this surface diffusion boundary connects.
Syntax:
getPatches()
Arguments: None
Return: list<steps::wm::Patch, length = 2>
- property id
Identifier string of the surface diffusion boundary.
- isBarInside(bars)
Returns a list of Booleans describing if bars are assigned to the surface diffusion boundary.
Syntax:
isBarInside(bars)
Arguments: list<index_t> bars
Return: list<bool, length = length(bars)>
- property patches
Reference to two steps.tetmesh.Patch patches connected by this surface diffusion boundary.
- setID(id)
Set the identifier string of the surface diffusion boundary.
Syntax:
setID(name)
Arguments: string name
Return: None
- this
An identification of the underlying cpp object
- class Memb(id, container, patches, verify=False, opt_method=1, search_percent=100.0, opt_file_name='', supplementary_comps=[])[source]
This class provides annotation for a group of triangles that comprise a surface describing a membrane in a Tetmesh. This may be the same description of one or several TmPatches in order for voltage-dependent transitions, currents and so on to be inserted in the membrane. A Memb object must be available if membrane potential calculation is to be performed.
Construction:
memb = steps.geom.Memb(id, container, patches, verify = False, opt_method=1, opt_file_name = ‘’, supplementary_comps=[])
Construct a Memb object with identifier string id and assign container as the parent geometry container. Set the collection of triangles in the membrane to those that belong to all TmPatches in patches. Perform some checks on suitability of membrane if verify is True: these checks will print warnings if membrane forms an open surface or if any triangle is found to have more than 3 neighbours. Specify optimization method with opt_method (default = 1): 1 = principal axis ordering (quick to set up but usually results in slower simulation than method 2). 2 = breadth first search (can be time-consuming to set up, but usually faster simulation. If 2:breadth first search is chosen then argument search_percent can specify the number of starting points to search for the lowest bandwidth. If a filename (with full path) is given in optional argument opt_file_name the membrane optimization will be loaded from file, which was saved previously for this membrane with solver method steps.solver.Tetexact.saveMembOpt()
By default STEPS only adds the inner compartments of the patches to the conduction volume, if other compartments should also be added to the conduction volume, they should be supplied through the supplementary_comps keyword parameter.
Arguments: string id steps.geom.Tetmesh container list<steps.geom.TmPatch> patches bool verify (default = False) int opt_method (default = 1) float search_percent (default=100) string opt_file_name (default = ‘’) list<steps.geom.TmComp> supplementary_comps
- countTris()
Returns the number of triangles assigned to the membrane.
Syntax:
countTris()
Arguments: None
Return: int
- countVerts()
Returns the number of vertices in the conduction volume and membrane surface.
Syntax:
countVertices()
Arguments: None
Returns: uint
- countVirtTris()
Returns the number of virtual triangles for the membrane forming a closed surface.
Syntax:
countTris()
Arguments: None
Return: uint
- countVolTets()
Returns the number of tetrahedrons assigned to the conduction volume.
Syntax:
countVolTets()
Arguments: None
Return: uint
- getAllTriIndices()
Returns a list of indices of all triangles assigned to the membrane.
Syntax:
getAllTriIndices()
Arguments: None
Return: list<index_t>
- getAllVertIndices()
Returns a list of all vertices in the conduction volume.
Syntax:
getAllVertices()
Arguments: None
Return: list<index_t>
- getAllVirtTriIndices()
Returns a list of all virtual triangles for the membrane forming a closed surface.
Syntax:
getAllVirtTris()
Arguments: None
Return: list<index_t>
- getAllVolTetIndices()
Returns a list of indices of all tetrahedrons assigned to the conduction volume.
Syntax:
getAllVolTetIndices()
Arguments: None
Return: list<index_t>
- getContainer()
Returns a reference to the parent steps.geom.Tetmesh container object.
Syntax:
getTetmesh()
Arguments: None
Return: steps.tetmesh.Tetmesh
- getID()
Get the identifier string of the membrane.
Syntax:
getID()
Arguments: None
Return: string
- isTriInside(tri)
Returns a list of Booleans describing if triangles tris are assigned to the membrane.
Syntax:
isTriInside(tris)
Arguments: list<index_t> tris
Return: list<bool, length = length(tris)>
- open()
Returns whether a membrane is open or not, that is whether it contains any holes or not.
Syntax:
open()
Arguments: None
Returns: bool
- this
An identification of the underlying cpp object
- property tris
List of indices of triangles associated to the membrane.